This documentation explain how to use the decorator for tasks, protocols, resources and views. The decorator allows standards python class and method to be referenced in the Constellab
ecosystem as tasks, protocols, resources or views.
Here are the main settings for the decorator:
- unique_name: string. For tasks, protocols and resources only. This name must be unique for the object type in the brick. It is used to instantiate the object. Once it is defined IT MUST NOT BE MODIFIED. If modified, the object will be considered as a new one, and the previous one will be deleted.
- human_name: string. This is the name people will see in the interface. It should be short.
- short_description: string. This is a brief explanation of the object that appears in the interface. It must be less than 255 characters long.
- hide: bool. If this is set to true, the object won't show up in the interface. This is helpful for objects that don't need to be seen in the interface, like abstract classes.
- style: TypingStyle. This sets the appearance of the object. See the "Style" bellow for more information
- deprecated:
TypingDeprecated
. Provide if the object is deprecated and should not be used anymore. Provide the deprecated_since
and deprecated_message
.
Style
This part sets the icon, icon color, and background color for the object when it appears in the interface. You can find the list of available icons here: https://constellab.community/icon.
Here is an exmaple of the style used in the playground
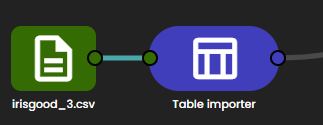
There are three types of icons to choose from.
Material icon
All the material icons are supported, you can find the list here : https://fonts.google.com/icons?icon.set=Material+Icons.
To use it, select the icon you want and copy the code (lowercase). Then, use TypingStyle.material_icon
. Here is an example on how to define the style :
from gws_core import resource_decorator, TypingStyle, TypingIconColor
@resource_decorator("Table", human_name="Table", short_description="2d excel like table",
style=TypingStyle.material_icon(material_icon_name="table_chart",
background_color="#413ebb",
icon_color=TypingIconColor.WHITE)
)
Community icon
The community icon are referenced here : https://constellab.community/icon. It supports custom color for the icon. In community they are referenced with the icon type : ICON.
To use it, select the icon you want and copy the technical name. Then, use TypingStyle.community_icon
. Here is an example on how to define the style :
from gws_core import resource_decorator, TypingStyle, TypingIconColor
@resource_decorator("Table", human_name="Table", short_description="2d excel like table",
style=TypingStyle.community_icon(icon_technical_name="table_icon",
background_color="#413ebb",
icon_color=TypingIconColor.WHITE)
)
Community image
The community icon are referenced here : https://constellab.community/icon. In community they are referenced with the icon type : IMAGE.
To use it, select the icon you want and copy the technical name. Then, use TypingStyle.community_image
. Here is an example on how to define the style :
from gws_core import resource_decorator, TypingStyle, TypingIconColor
@resource_decorator("Table", human_name="Table", short_description="2d excel like table",
style=TypingStyle.community_image(icon_technical_name="table_image",
background_color="#413ebb"))
Task style
For the task, if the style is not defined it is automatically set based on resources. It takes the style of the first input resource type or the first output resource type. It copies the icon, background color and icon color if it not defined in the task decorator.
If you want you task to use the same style as the input resource, then don't define the style on the task and it will be set automatically.
You can also copy the style of a resource with the possibility to override some values. This can be useful to use the color of a resource for you task but override the icons. To do this use the copy_style
class method of Resource
.
Here is an example of a copy with the icon overridden (it copies the color of the File
class).
style=File.copy_style(icon_technical_name='folder_zip', icon_type=TypingIconType.MATERIAL_ICON)